LXI SOAP Interface
What is SOAP?
DEFINITION -
SOAP (Simple Object Access Protocol) is a way for a program running in one kind of operating system (such as Windows 2000) to communicate with a progam in the same or another kind of an operating system (such as Linux) by using the World Wide Web's Hypertext Transfer Protocol (HTTP)and its Extensible Markup Language (XML) as the mechanisms for information exchange. Since Web protocols are installed and available for use by all major operating system platforms, HTTP and XML provide an already at-hand solution to the problem of how programs running under different operating systems in a network can communicate with each other.
SOAP specifies exactly how to encode an HTTP header and an XML file so that a program in one computer can call a program in another computer and pass it information. It also specifies how the called program can return a response.
SOAP was developed by Microsoft, DevelopMentor, and Userland Software and has been proposed as a standard interface to the Internet Engineering Task Force (IETF). It is somewhat similar to the Internet Inter-ORB Protocol (IIOP), a protocol that is part of the Common Object Request Broker Architecture (CORBA). Sun Microsystems' Remote Method Invocation (RMI) is a similar client/server interprogram protocol between programs written in Java.
An advantage of SOAP is that program calls are much more likely to get through firewall servers that screen out requests other than those for known applications (through the designated port mechanism). Since HTTP requests are usually allowed through firewalls, programs using SOAP to communicate can be sure that they can communicate with programs anywhere.
Pickering LXI Devices
Pickering LXI devices contains two SOAP services (Web services). The first, picoms, is for getting fundamental information about the LXI device and establishing a connection to the device. The second, picards, is for controlling switch cards inside the LXI device. These services are defined in a pair of WSDL (Web Service Description Language) files which may be used to create an interface. The methodology for this process varies from language to language, refer to the SOAP documentation for the language being used.
The following section refers to the use of SOAP from the Perl environment.
Pickering Interfaces provides two basic Perl modules/packages for these two SOAP services named picoms.pm and picards.pm.
Use of the Picoms.pm Package
1. Load the Picoms library module
2. Create an object of type Picoms
3. Set fundamental web service parameters like the endpoint of the service and timeout using the SoapSettings method (default timeout is 10s)
4. Then use the interface
For example:
$lxi-> use Pickering::Lxi::Picoms;
$lxi1 = new Pickering::Lxi::Picoms;
$lxi1 -> SoapSettings("http://192.168.10.64/bin/picoms",20);
@res = $lxi1 -> SbVersion();
print "\nLXI device uses Server Bridge at version ". $res[1]/100;
Echo();
Note that variable $lxi1 is an object, so that you can change fundamental soap setting and you can connect to another LXI device or you can a more similar object in one time if you want.
Using Picards is similar. There is only one important step after step 3): before you call any control functions you have to set the location of the card using function SetCardLocation(bus, slot) because most functions expect card location. After that you can use all functions of Picards package.
The interface is heavily based on the Pickering PXI interfaces pilpxi.dll. However, since Card location is always sent by SOAP header, the parameters CardNum is not used. Another notable difference is that this is a SOAP service, there is no session variable. With the Pickering SOAP interface one doesn't open a card and then control the bits of the card and finally close the card when finished. Instead almost every service's method will open the card, do the command and then close the card.
use Pickering::Lxi::Picards;
$lxi1 = new Pickering::Lxi::Picards;
$lxi1 -> SoapSettings("http://192.168.10.91/bin/picards",20);
$lxi1 -> SetCardLocation(2,9);
@res = $lxi1 -> Status();
print "\nStatus of the card is: $res[1]";
Almost every function returns an array (except for example ErrorCodeToMessage()). The first item of the array is always retun code (int). If return code is 0 then operation was successful, any other value represents an error. Please see documentation for Client Bridge.
Next item in returned arrays represent values which are returned by function. It can be one value or array or reference to hash. Please, read manual for every function.
You can create your own libraries to cooperate with our soap services and WSDL files are available to assist this process. Our own libraries are based on the SOAP::Lite PERL package.
In general most programs will only need to use Picards, this contains all the card control functions. Picoms concerns itself with communications and card identity functions.
Quick Synopsis of Pickering Switch Card Interface
A Pickering switch card presents an interface composed of a series of one or more sub-units. Each sub-unit represents a set of identical input or output objects, usually switches.
Take the example of a 40-677 Multiplexer card. This has two output sub-units, the first a 20 wide multiplexer, the second a single bit wide simple switch.
The driver module interface provides a set of methods to manipulate these sub-units.
Example Program
#!/usr/bin/perl #============================================================= # Example for 41-751 + 40-677 Mux # Created by: Alan Hume, (c) Pickering Interfaces Ltd., 2007 #============================================================= use Pickering::Lxi::Picards; print "========================================\n"; print " EXAMPLE: Using Perl package Picards \n"; print " (c) Pickering Interfaces Ltd., 2007 \n"; print "========================================\n"; # ----------------------------- Main code -------------------------------------- # Define objects for each card in the rack $dev_battery_sim = new Pickering::Lxi::Picards; $dev_battery_sim -> SoapSettings("http://192.168.1.109/bin/picards",20); $dev_battery_sim -> SetCardLocation(2, 15); $dev_mux = new Pickering::Lxi::Picards; $dev_mux -> SoapSettings("http://192.168.1.109/bin/picards",20); $dev_mux -> SetCardLocation(2, 14); print "\n\n------------- CARD INFORMATION--------------\n"; print "\nCard ID:\n"; @res = $dev_battery_sim -> CardId(); print "Device id = $res[1]\n"; PSU_On(); Set_Enable_Channel( 12 ); Set_Volts(3.5); PSU_Off(); print "\n\n == THE END ==\n"; # PSU Output enable is single bit at sub-unit 8 sub PSU_On { print "Turn PSU on\n"; my @data = (1); @res = $dev_battery_sim -> WriteSub(8, @data); } # PSU Output enable is single bit at sub-unit 8 sub PSU_Off { print "Turn PSU off\n"; my @data = (0); @res = $dev_battery_sim -> WriteSub(8, @data); } # PSU Voltage is 16 bit register at sub-unit 3 # 0xFFFFFFF == 6V sub Set_Volts { my @data = (int($_[0] * 65535 / 6)); print "Set voltage $_[0] V == $data[0] binary\n"; @res = $dev_battery_sim -> WriteSub(3, @data); } sub Set_Enable_Channel { my $channel = @_[0]; $dev_mux -> OpBit(1, $channel, 1); $dev_mux -> OpBit(2, 1, 1); }
Use with .NET
The following example uses SharpDevelop, a public domain software development tool for the C# language. It is available from the SourceForge web site, http://sourceforge.net/projects/sharpdevelop .
SOAP is well supported in the .NET framework and creating SOAP applications is very very simple. The following example shows how to create a small C# application which can communicate with our SOAP server. This can be done in one minute, here's how:
(1) Create a new C# console application.
(2) Add new web reference (right click on references in Projects view). This action opens dialog. Use the HTTP GET request to get a WSDL file from the Pickering LXI device. For example: http://192.168.10.50/bin/picoms?wsdl .
In WSDL tab you can see the WSDL document of the picoms web service (SOAP service).
(3) Change the reference name to your own name, for example to LxiPiComs and namespace to SOAPTest.LxiPiComs, see picture:
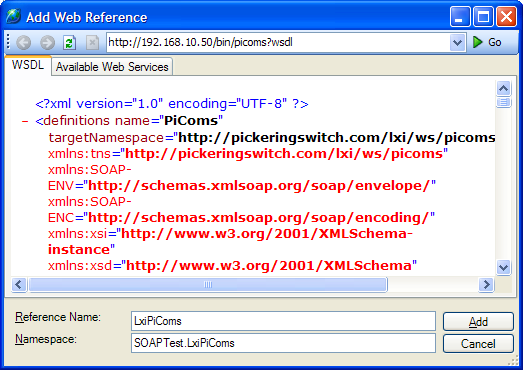
The tab "Available Web Services" shows classes and methods of the web service:
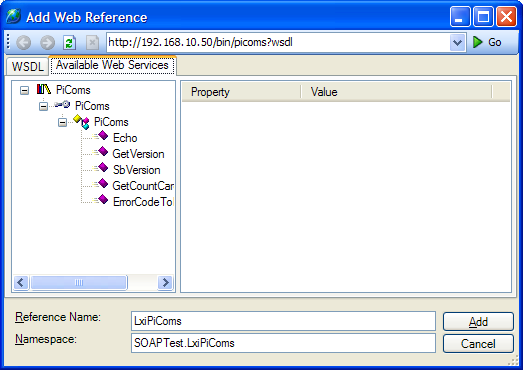
(4) Add this reference to your C# console project.
(5) Add namespace "SOAPTest.LxiPiComs" to your source code by statement "using SOAPTest.LxiPiComs;" and your code which uses this web service, for example:
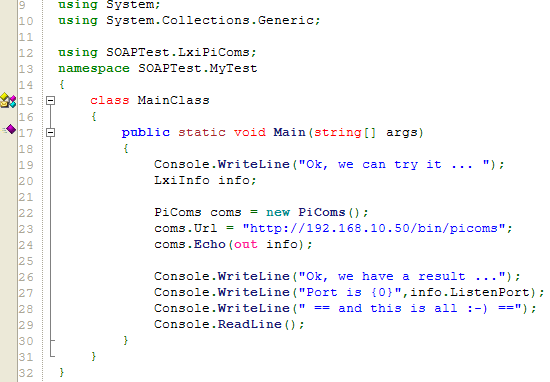
Note: Don't forget set up correct URL to the web service in your source code!
(6) Build and run the project. See result:
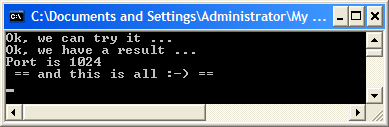
That really is all there is to it